오늘이라도
[Raspberry Pi] 3. 파이썬 기초 : 내장 함수, 형변환, len, 조건문, 반복문, range(), time.sleep(), 함수 작성 / Hello World, LED 켜기, 깜빡이기 본문
취업성공패키지 SW 개발자 교육/사물 인터넷(IoT)
[Raspberry Pi] 3. 파이썬 기초 : 내장 함수, 형변환, len, 조건문, 반복문, range(), time.sleep(), 함수 작성 / Hello World, LED 켜기, 깜빡이기
upcake_ 2020. 7. 8. 10:47반응형
https://github.com/upcake/Class_Examples
교육 중에 작성한 예제들은 깃허브에 올려두고 있습니다.
gif 파일은 클릭해서 보는 것이 정확합니다.
- 파이썬 기초 : 내장 함수(upper, lower, count, swapcase, replace, split), 형변환, len -
#builtin function
#upper, lower, count, swapcase
a = "Good morning, Man"
print(a.upper())
print(a.lower())
print(a.count('o'))
print(a.swapcase())
#replace
a = "Life is too short"
print(a.replace("Life", "Your height"))
#split
a = "I regret what I did to you"
print(a.split())
d = "home, mother, sweet"
print(d.split(','))
print(d.split(","))
#casting
n = int('123') #string > number
f = float("365.7") #string > float
s = str(123) #number > string
ss = str(456) + "789"
print(n)
print(f)
print(s)
print(ss)
#len
print(len("hi"))
▲내장 함수, 형변환, len
>>> %Run 200708_1.py
GOOD MORNING, MAN
good morning, man
3
gOOD MORNING, mAN
Your height is too short
['I', 'regret', 'what', 'I', 'did', 'to', 'you']
['home', ' mother', ' sweet']
['home', ' mother', ' sweet']
123
365.7
123
456789
2
>>>
▲실행 결과
- 파이썬 기초 : 조건문(if, while), 반복문(for), range(), time.sleep() -
#if
name = 'jane'
if name == 'jane':
print("name is jane")
else :
print("name is not jane")
age = 35
if age > 30 :
print('more than 30')
else :
print('less than 30')
a = 5
if a == 5:
print ('True')
else:
print ('False')
if a > 3 and a < 10:
print ('True')
else:
print ('False')
#elif
if a == 4:
print ('number is 4')
elif a < 10:
print ('number less than 10')
else:
print ('number is something')
#while
a = 0
while a < 10:
a = a + 1
print (a)
#for
odd_number = [1, 3, 5, 7, 9, 11, 13]
for num in odd_number:
print(num)
number = [1, 2, 3, 4, 5, 6, 7]
for num in number:
if num % 2 == 0:
print(num)
#range()
range(10) #0 ~ 9
range(5, 10) #5 ~ 9
range(10, 20, 2) #10, 12, 14, 16, 18
for i in range(10):
print(i)
#gugudan
for x in range(2, 10):
print("-------[" + str(x) + "dan]--------")
for y in range(1, 10):
print(x, " X ", y, " = ", x*y)
print("---------------------")
#time
#sleep(second)
import time
for i in range(10):
print(i)
time.sleep(0.1)
▲조건문, 반복문, range(), time.sleep()
>>> %Run 200708_2.py
name is jane
more than 30
True
True
number less than 10
1
2
3
4
5
6
7
8
9
10
1
3
5
7
9
11
13
2
4
6
0
1
2
3
4
5
6
7
8
9
-------[2dan]--------
2 X 1 = 2
2 X 2 = 4
2 X 3 = 6
2 X 4 = 8
2 X 5 = 10
2 X 6 = 12
2 X 7 = 14
2 X 8 = 16
2 X 9 = 18
---------------------
-------[3dan]--------
3 X 1 = 3
3 X 2 = 6
3 X 3 = 9
3 X 4 = 12
3 X 5 = 15
3 X 6 = 18
3 X 7 = 21
3 X 8 = 24
3 X 9 = 27
---------------------
-------[4dan]--------
4 X 1 = 4
4 X 2 = 8
4 X 3 = 12
4 X 4 = 16
4 X 5 = 20
4 X 6 = 24
4 X 7 = 28
4 X 8 = 32
4 X 9 = 36
---------------------
-------[5dan]--------
5 X 1 = 5
5 X 2 = 10
5 X 3 = 15
5 X 4 = 20
5 X 5 = 25
5 X 6 = 30
5 X 7 = 35
5 X 8 = 40
5 X 9 = 45
---------------------
-------[6dan]--------
6 X 1 = 6
6 X 2 = 12
6 X 3 = 18
6 X 4 = 24
6 X 5 = 30
6 X 6 = 36
6 X 7 = 42
6 X 8 = 48
6 X 9 = 54
---------------------
-------[7dan]--------
7 X 1 = 7
7 X 2 = 14
7 X 3 = 21
7 X 4 = 28
7 X 5 = 35
7 X 6 = 42
7 X 7 = 49
7 X 8 = 56
7 X 9 = 63
---------------------
-------[8dan]--------
8 X 1 = 8
8 X 2 = 16
8 X 3 = 24
8 X 4 = 32
8 X 5 = 40
8 X 6 = 48
8 X 7 = 56
8 X 8 = 64
8 X 9 = 72
---------------------
-------[9dan]--------
9 X 1 = 9
9 X 2 = 18
9 X 3 = 27
9 X 4 = 36
9 X 5 = 45
9 X 6 = 54
9 X 7 = 63
9 X 8 = 72
9 X 9 = 81
---------------------
0
1
2
3
4
5
6
7
8
9
>>>
▲실행 결과
- 파이썬 기초 : 함수 작성 -
#function
#no return
def MySum1():
a = 10
b = 20
print (a+b)
MySum1()
#yes return
def MySum2():
a = 10
b = 20
return (a+b)
print (MySum2())
#yes parameter
def MySum3(a, b):
return (a + b)
print(MySum3(20, 30))
print(MySum3("aaa", "AAA"))
#global variable
a = 100
def MySum4(b):
global a
a = 200
return (a + b)
print(MySum4(300))
#import modulename as alias
#import time
#import RPi.GPIO as GPIO
#import math
#import random
#Save Python file as ~~~~~~.py >> ~~~~~~ : module name
#can call > ~~~~~~.variable / ~~~~~~.function
▲함수 작성
>>> %Run 200708_3.py
30
30
50
aaaAAA
500
>>>
▲실행 결과
- Hello World, LED 점등, 깜빡이기 -
print('Hello')
print('World')
import random
for x in range(1, 10):
random_number = random.randint(1, 6) #1 ~ 6
print(random_number)
▲1.Hello World.py
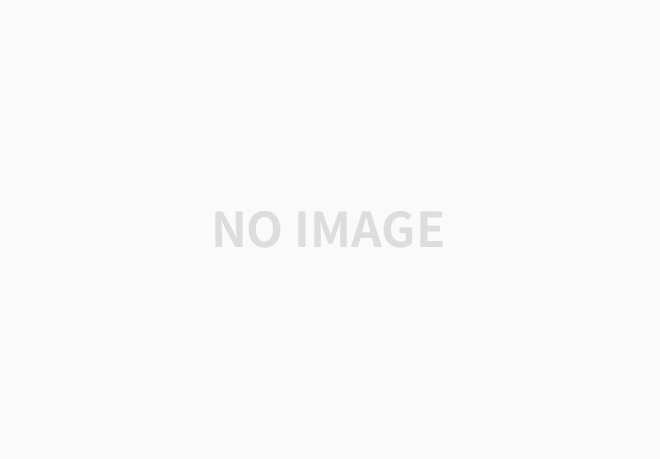
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM) #BCM : 확장보드의 이름대로 핀번호를 준다
GPIO.setup(4, GPIO.OUT, initial=GPIO.LOW)
#7번 핀을 OUT (출력) 목적으로 사용하며 초기 값은 LOW 상태로 시작하도록 설정함
GPIO.output(4, GPIO.HIGH)
#4번 핀에 HIGH 상태로 출력함
time.sleep(3)
GPIO.cleanup()
#GPIO핀들을 개방시켜줌, 개방시키지 않고 종료하면 다음 실행 시 경고 메시지가 표시됨
▲2.LED.py
import RPi.GPIO as GPIO #GPIO 모듈 불러오기
import time
GPIO.setmode(GPIO.BCM) #GPIO 옆 번호를 사용
#4 > GPIO04 => 핀번호 7번 (다음부터는 BOARED로 사용)
GPIO.setup(4, GPIO.OUT, initial = GPIO.LOW)
try:
while (True):
GPIO.output(4, GPIO.HIGH)
time.sleep(0.5)
GPIO.output(4, GPIO.LOW)
time.sleep(0.5)
except KeyboardInterrupt: #Ctrl + C로 종료
GPIO.cleanup()
▲3.LED Blink.py
반응형
'취업성공패키지 SW 개발자 교육 > 사물 인터넷(IoT)' 카테고리의 다른 글
[Raspberry Pi] 5. 버튼 눌러서 LED 시간 증가 (0) | 2020.07.10 |
---|---|
[Raspberry Pi] 4. LED 조작 : PWM, 버튼 이용 (0) | 2020.07.09 |
[Raspberry Pi] 2. 라즈베리 파이 설정 : 원격 데스크톱, 삼바 / 리눅스 기초 명령어 / 파이썬 기초 (0) | 2020.07.07 |
[Raspberry Pi] 1. 라즈베리 파이 개발 환경 구축 (0) | 2020.07.06 |
[Arduino] 8. RC카로 풍선 터뜨리기 (0) | 2020.04.29 |